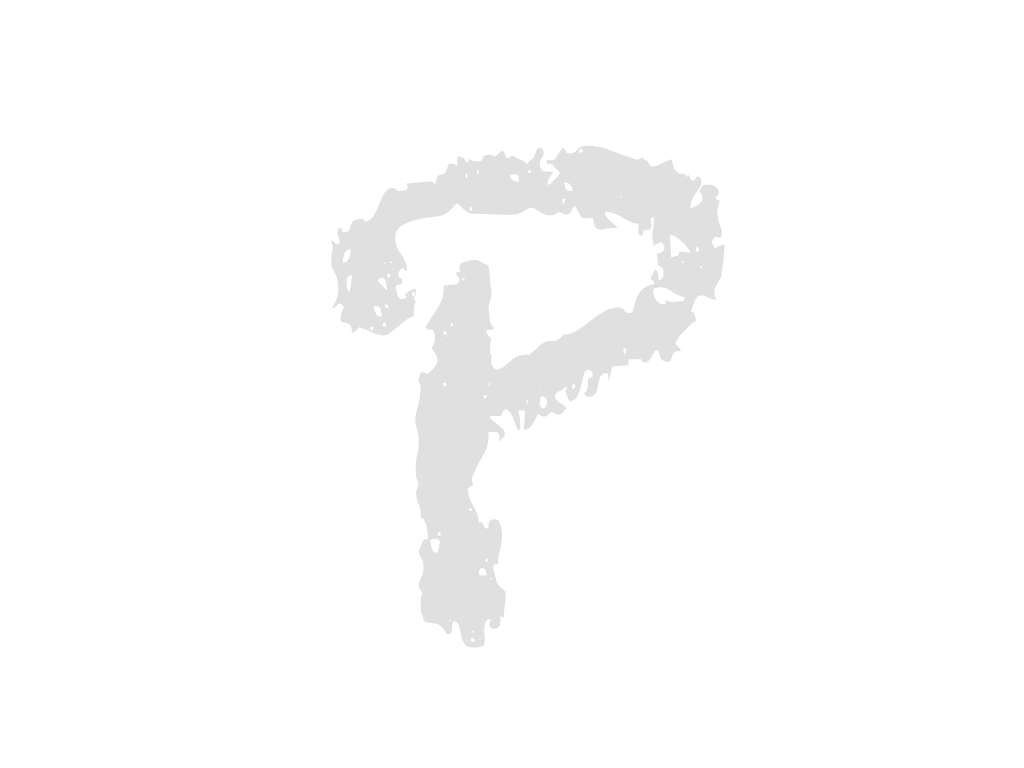
+++ random_data_push.py
... | ... | @@ -0,0 +1,60 @@ |
1 | +import time, sys, requests, json | |
2 | +#import board | |
3 | +#import adafruit_dht | |
4 | +import random | |
5 | + | |
6 | +# 데이터 핀 3번을 사용면, GPIO2로 설정 | |
7 | +#pin = board.D2 | |
8 | +#dhtDevice = adafruit_dht.DHT11(pin) | |
9 | + | |
10 | +# EdgeX server ip | |
11 | +edgexip = "192.168.1.191" | |
12 | + | |
13 | +while True: | |
14 | + try: | |
15 | + # 온도 및 습도 값을 읽어옴 | |
16 | + | |
17 | + #온도 | |
18 | + #temperature_c = dhtDevice.temperature | |
19 | + temperature_c = random.uniform(35, 40) | |
20 | + temperature_f = temperature_c * (9 / 5) + 32 | |
21 | + | |
22 | + #습도 | |
23 | + #humidity = dhtDevice.humidity | |
24 | + humidity = random.uniform(20, 80) | |
25 | + | |
26 | + print( | |
27 | + "온도: {:.1f} F / {:.1f} C 습도: {}% ".format( | |
28 | + temperature_f, temperature_c, humidity | |
29 | + ) | |
30 | + ) | |
31 | + | |
32 | + if temperature_c is not None and humidity is not None : | |
33 | + urlTemp = 'http://%s:49986/api/v1/resource/Temp_and_Humidity_sensor_cluster_01/temperature' % edgexip | |
34 | + urlHum = 'http://%s:49986/api/v1/resource/Temp_and_Humidity_sensor_cluster_01/humidity' % edgexip | |
35 | + | |
36 | + headers = {'content-type': 'application/json'} | |
37 | + response = requests.post(urlTemp, data=json.dumps(int(temperature_c)), headers=headers,verify=False) | |
38 | + response = requests.post(urlHum, data=json.dumps(int(humidity)), headers=headers,verify=False) | |
39 | + | |
40 | + | |
41 | + | |
42 | + | |
43 | + else: | |
44 | + print('Read error') | |
45 | + time.sleep(100) | |
46 | + | |
47 | + | |
48 | + except RuntimeError as error: | |
49 | + # 오류 발생 시 계속 진행 | |
50 | + print(error.args[0]) | |
51 | + time.sleep(2.0) | |
52 | + continue | |
53 | + except Exception as error: | |
54 | + # 예기치 않은 오류 시 종료 | |
55 | + # dhtDevice.exit() | |
56 | + print("Unexpected error:", error) | |
57 | + sys.exit() | |
58 | + raise error | |
59 | + | |
60 | + time.sleep(2.0) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?